How to Automatically Update Product Prices Based on Stock Levels in WooCommerce
Getting your Trinity Audio player ready...
|
How to Automatically Update Product Prices Based on Stock Levels in WooCommerce
Managing a WooCommerce store can be both rewarding and challenging, especially when it comes to pricing strategies. One powerful technique to optimize your sales and profits is adjusting your product prices based on stock levels. This method allows you to implement a dynamic pricing strategy, ensuring that prices respond to inventory changes in real time. In this article, we will walk you through how to automatically update product prices based on stock levels in WooCommerce using PHP code. We will also cover why this method is beneficial for WooCommerce developers and business owners.
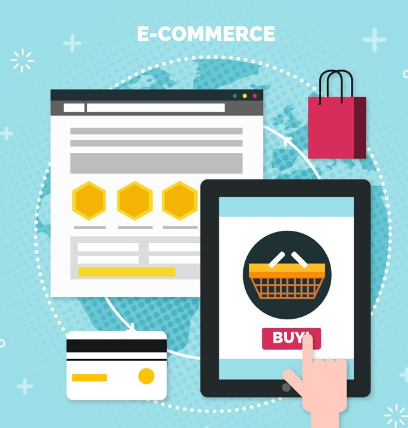
Why Adjust Prices Based on Stock Levels?
Before diving into the technical details, it’s important to understand the benefits of automatically adjusting prices based on stock availability:
- Boost Sales During Overstock: If you have excess stock, lowering prices can help clear inventory faster.
- Maximize Profits During Low Stock: When stock is running low, raising prices can increase profit margins while maintaining demand.
- Encourage Urgency: Low stock levels combined with higher prices can create a sense of scarcity, prompting customers to make quicker purchasing decisions.
- Reduce Deadstock: Overstock can lead to unsold items. A dynamic pricing strategy helps avoid this problem by encouraging sales through price reductions.
Now that we know the benefits, let’s dive into the code that will help you implement this strategy in WooCommerce.
Prerequisites
Before implementing this solution, make sure you meet the following requirements:
- A WooCommerce-powered WordPress website.
- Basic understanding of PHP and how WooCommerce hooks work.
- Access to your theme’s
functions.php
file or the ability to create a custom plugin.
Steps to Automatically Update Prices Based on Stock Levels
1. Understanding the Logic
The idea behind dynamic pricing based on stock levels is simple:
- High Stock: Reduce the price to clear out inventory.
- Low Stock: Increase the price to boost profits.
- Medium Stock: Maintain standard pricing.
We will be using WooCommerce hooks to adjust product prices when the stock level changes. The stock levels can be divided into three categories:
- Low stock: A small number of products remaining (e.g., less than 10 items).
- Medium stock: Moderate availability (e.g., between 10 and 50 items).
- High stock: Plenty of stock available (e.g., more than 50 items).
2. Writing the PHP Code
Now, let’s move forward with the PHP code that dynamically updates product prices based on stock levels. This code can be added to your theme’s functions.php
file or a custom plugin.
// Hook into WooCommerce stock level update
add_action('woocommerce_product_set_stock', 'update_price_based_on_stock', 10, 1);
/**
* Automatically adjust product prices based on stock levels.
*
* @param WC_Product $product WooCommerce product object.
*/
function update_price_based_on_stock( $product ) {
// Get stock quantity
$stock_quantity = $product->get_stock_quantity();
// Define price adjustment thresholds
$low_stock_threshold = 10;
$high_stock_threshold = 50;
// Get current price
$regular_price = $product->get_regular_price();
// Logic to adjust prices based on stock levels
if ( $stock_quantity <= $low_stock_threshold ) {
// Low stock - increase price by 20%
$new_price = $regular_price * 1.20;
} elseif ( $stock_quantity >= $high_stock_threshold ) {
// High stock - decrease price by 10%
$new_price = $regular_price * 0.90;
} else {
// Medium stock - keep the price the same
$new_price = $regular_price;
}
// Update the product price if it has changed
if ( $new_price !== $regular_price ) {
$product->set_regular_price( $new_price );
$product->save(); // Save changes
}
}
/**
* Ensure the price is updated when stock is manually changed in admin.
*/
add_action('woocommerce_product_object_updated_props', 'update_price_based_on_stock_level', 10, 2);
function update_price_based_on_stock_level( $product, $updated_props ) {
if ( in_array( 'stock_quantity', $updated_props ) ) {
update_price_based_on_stock( $product );
}
}
3. How the Code Works
- Hook into WooCommerce’s Stock Update: We use the
woocommerce_product_set_stock
action hook to monitor when stock levels change. This hook triggers theupdate_price_based_on_stock
function. - Price Adjustment Logic: In the
update_price_based_on_stock
function, we check the product’s stock level and adjust the price accordingly:- If the stock is low (e.g., less than 10 units), the price is increased by 20%.
- If the stock is high (e.g., more than 50 units), the price is reduced by 10%.
- If the stock is in between, the price remains the same.
- Admin Interface Updates: We also ensure that if a store admin manually changes the stock levels in the WooCommerce dashboard, the price will update accordingly using the
woocommerce_product_object_updated_props
hook.
4. Testing the Code
After implementing the code, you need to test it to ensure it works as expected. Here’s how:
- Add a product to your WooCommerce store and manually adjust the stock levels.
- Observe how the price changes according to the stock levels you set.
- You can also simulate low and high stock levels by using test orders to trigger price updates automatically.
5. Adjusting the Price Multipliers
The code above uses a 20% increase for low stock and a 10% decrease for high stock. These values can be easily customized depending on your specific pricing strategy. Simply modify the multipliers in the code:
// Low stock - increase price by X% (e.g., 1.20 for 20%)
$new_price = $regular_price * 1.20;
// High stock - decrease price by Y% (e.g., 0.90 for 10%)
$new_price = $regular_price * 0.90;
Adjust the percentages according to your business model and product types.
SEO Considerations
When writing articles like this one for WooCommerce developers, it’s important to make sure the content is SEO-friendly. Here are a few tips:
- Keyword Optimization: The primary keyword for this article is “Automatically Update Product Prices Based on Stock Levels in WooCommerce.” This keyword should be used in the title, headers, and naturally throughout the text.
- Structured Data: Break the content into sections with clear headers to improve readability and SEO. Google favors well-structured content that is easy to understand and navigate.
- Internal and External Linking: Encourage developers to explore other related articles or resources, such as WooCommerce documentation, to improve the authority and relevance of your post.
Conclusion
Automatically updating product prices based on stock levels is a powerful tool that can help WooCommerce store owners optimize their pricing strategies, increase profits, and reduce deadstock. With the simple PHP code provided in this article, you can implement dynamic pricing directly in your store and customize it according to your business needs.
By leveraging this technique, you not only enhance your store’s performance but also provide a seamless, automated pricing solution that responds to real-time inventory changes. Happy coding!
For further assistance, Contact us